Blog
Learn frontend skills in-depth at Frontendly, where we dive into React, TypeScript, JavaScript, Algorithm, CSS, and more, all in a clear and practical fashion.
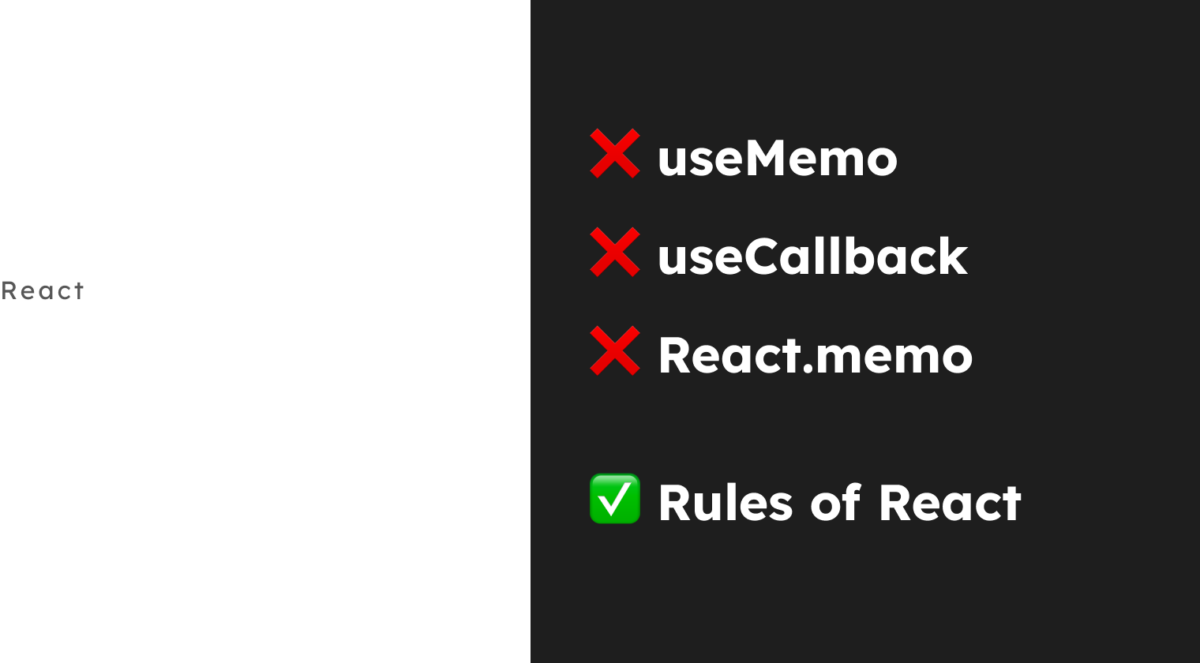
React Compiler – What, Why & How?
Discover how the React Compiler optimises apps by auto-memoizing code, eliminating the need for useMemo, useCallback, and React.memo, ensuring more efficient updates.
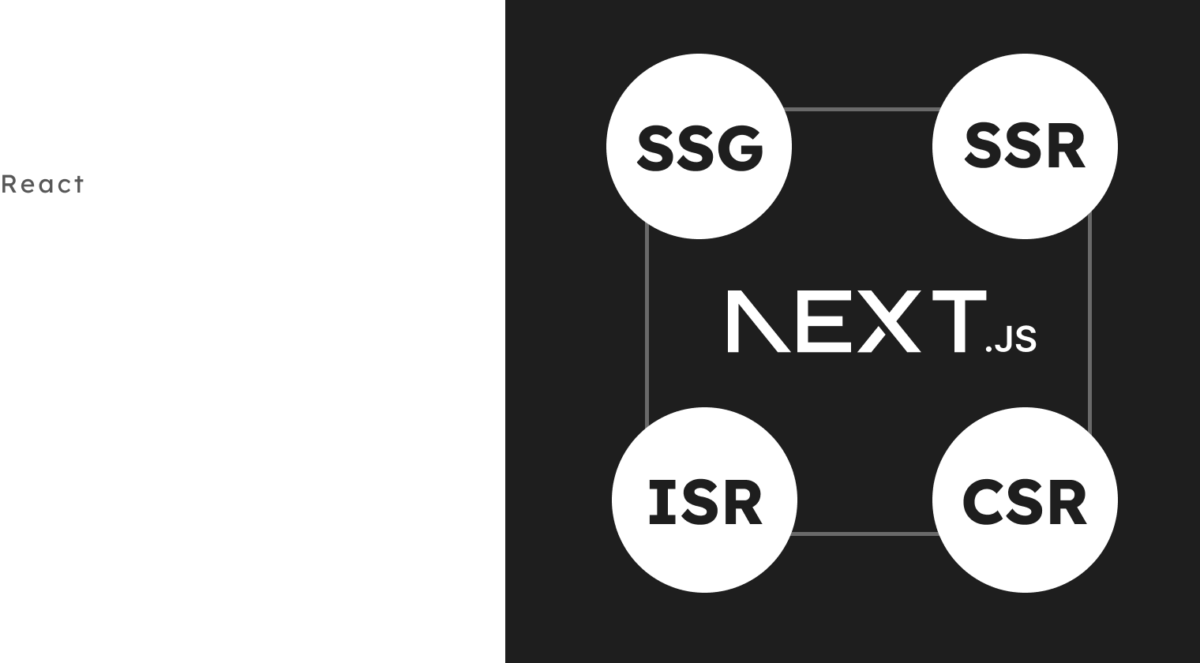
SSG, SSR, ISR, CSR Rendering Strategies in NextJS
Dive into how SSG, SSR, ISR, and CSR in NextJS can boost performance and efficiency.
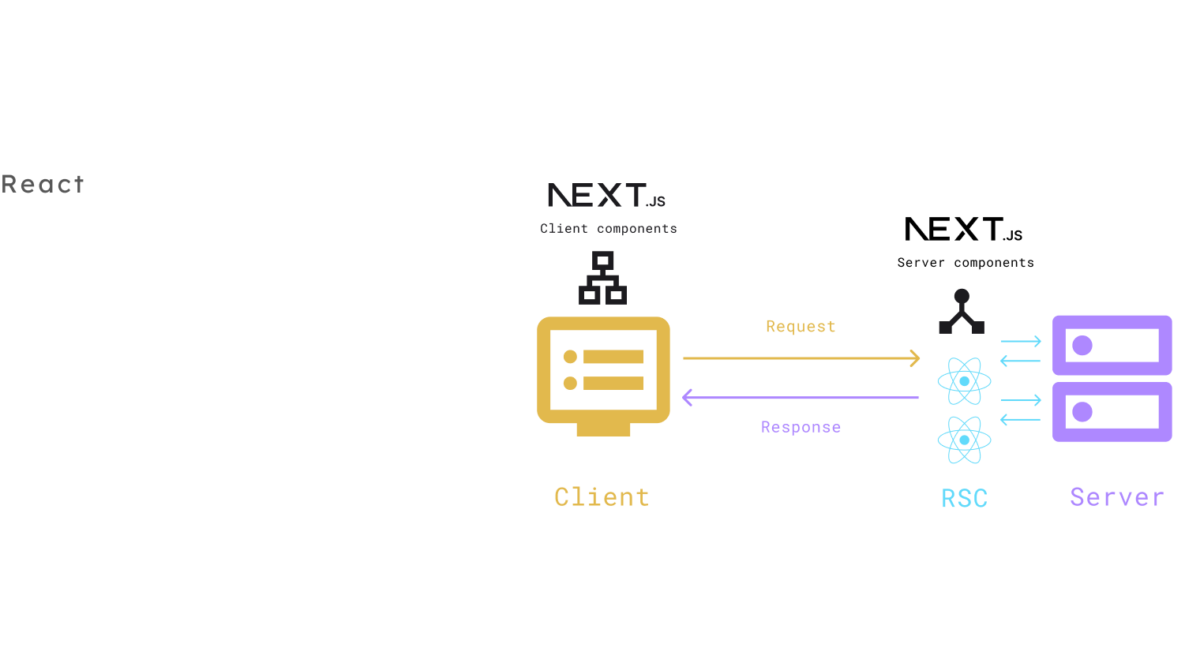
Server and Client Component – NextJS
Dive into NextJS’s server and client components: understanding their key features and collaborative functionality.
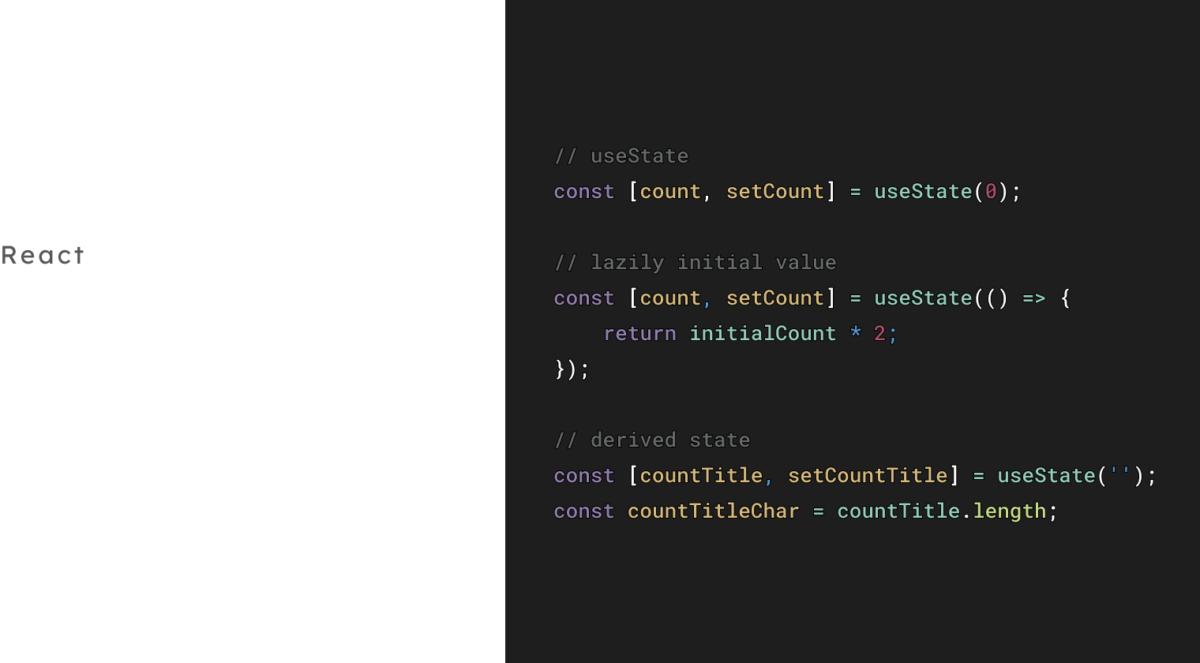
React useState: A Deep Dive into Hooks
Dive into React’s useState hook: master its uses and avoid common pitfalls.
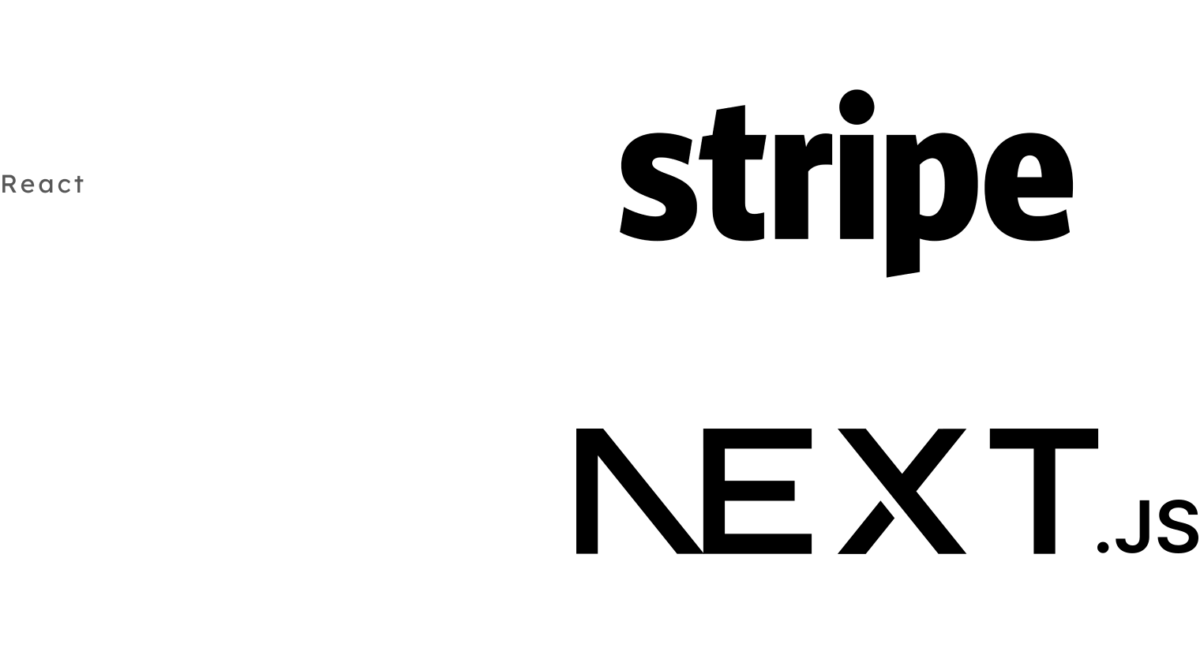
Testing Stripe Webhook Locally with Next.js
Learn how to test Stripe webhooks locally using Next.js, streamlining your integration process and accelerating application development
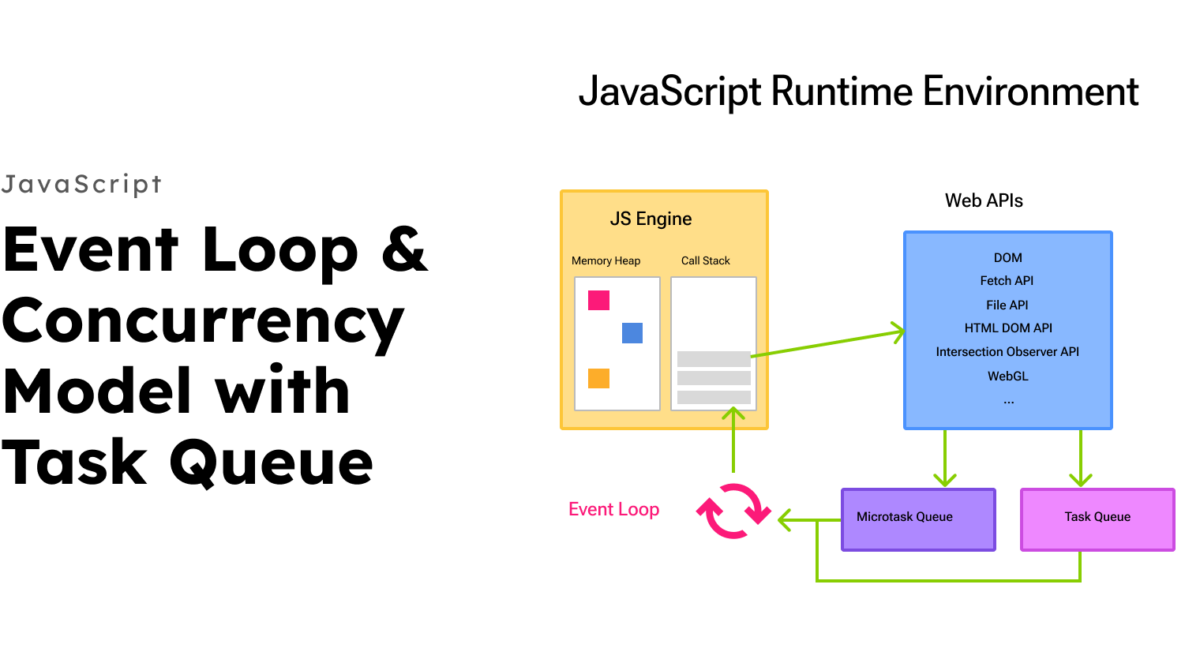
Event Loop & Concurrency Model – Task Queue – How the JS Engine Works – Part 3/6
Master JS async concurrency model with Web API, task queue, and event loop in the JS Runtime Environment through coding examples
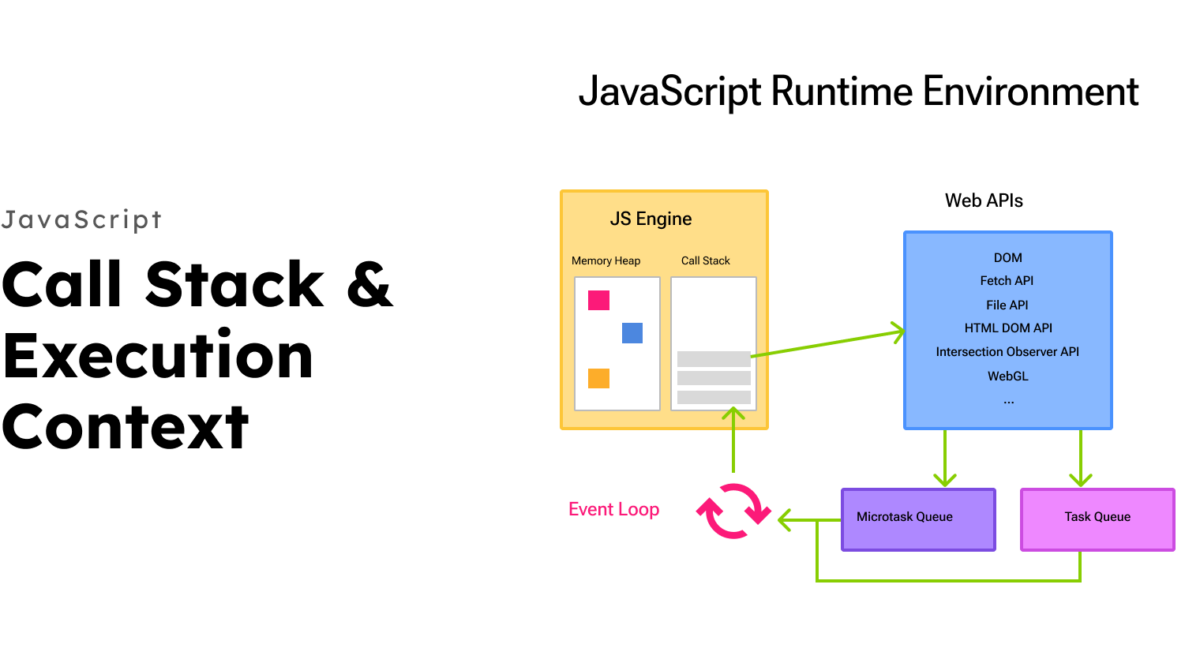
Call Stack & Execution Context – How the JS Engine Works – Part 2/6
Explore how the call stack and execution context function with clear, practical examples.
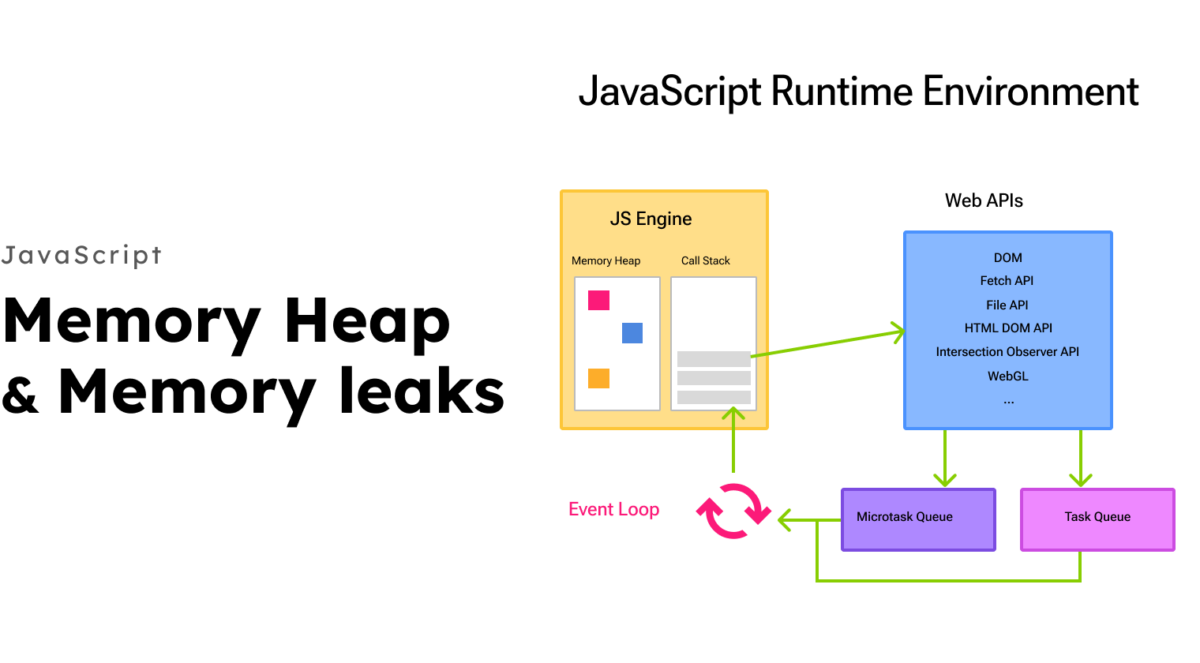
Understanding Memory Heap & Memory Leaks – How the JS Engine Works – Part 1/6
Learn JS synchronous nature, asynchronous programming, memory leaks and Memory Heap.
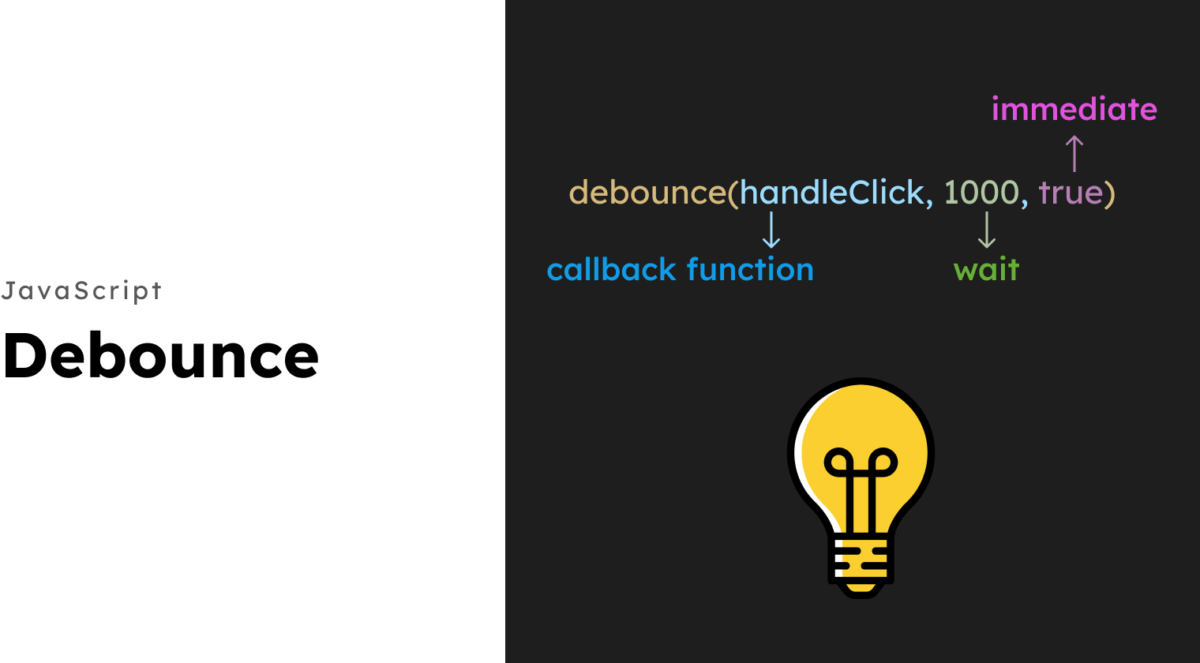
Debounce in JavaScript
Learn how to write your own debounce function, a crucial skill for your next JS interview.
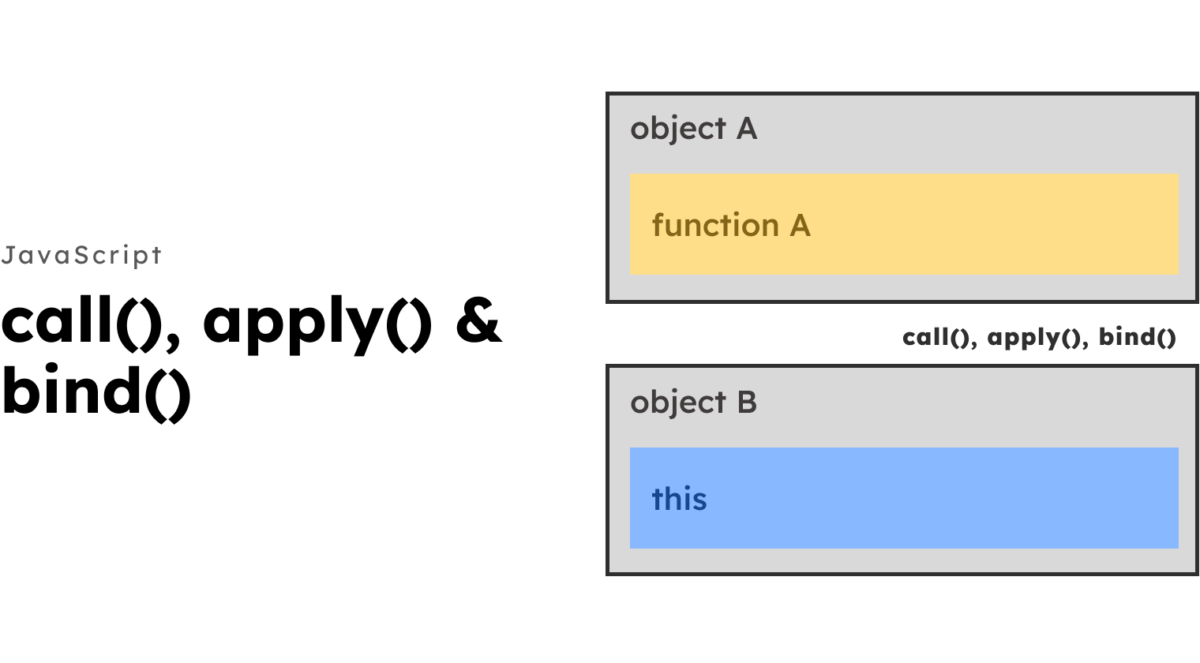
Understanding call(), apply() & bind() in JavaScript: Learn & Build Your Own
Explore JavaScript’s call(), apply(), and bind() methods: understand their functionality, differences, and learn to write your own.
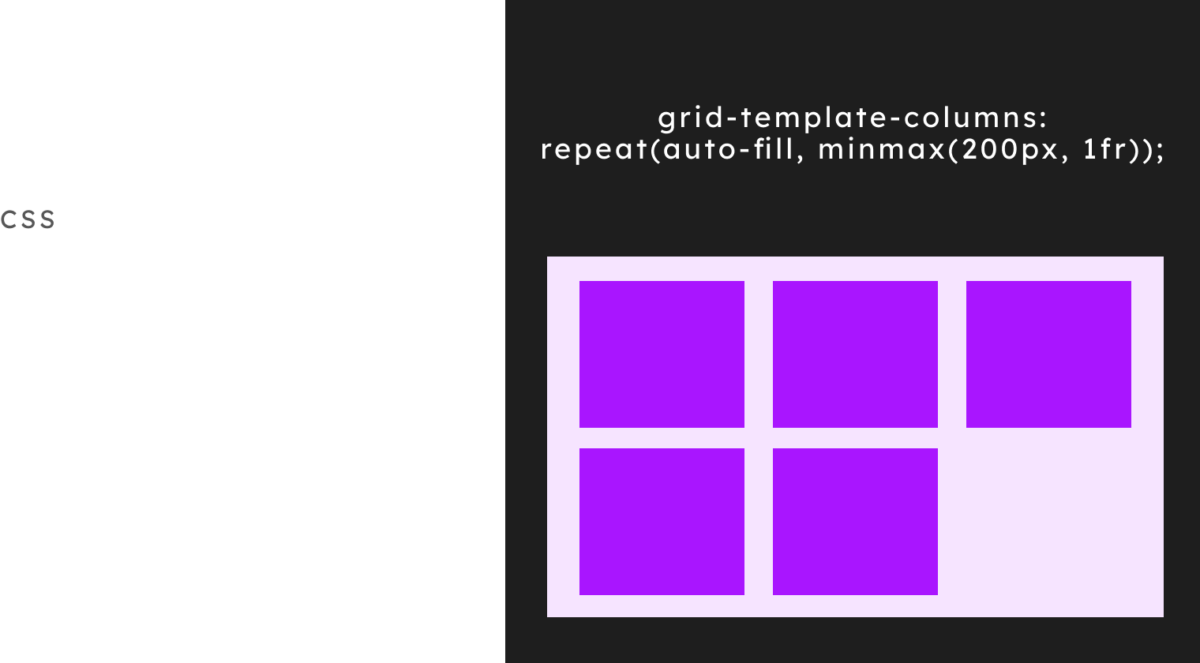
Responsive Card Grid Layout
Explore creating a responsive card grid with detailed examples.
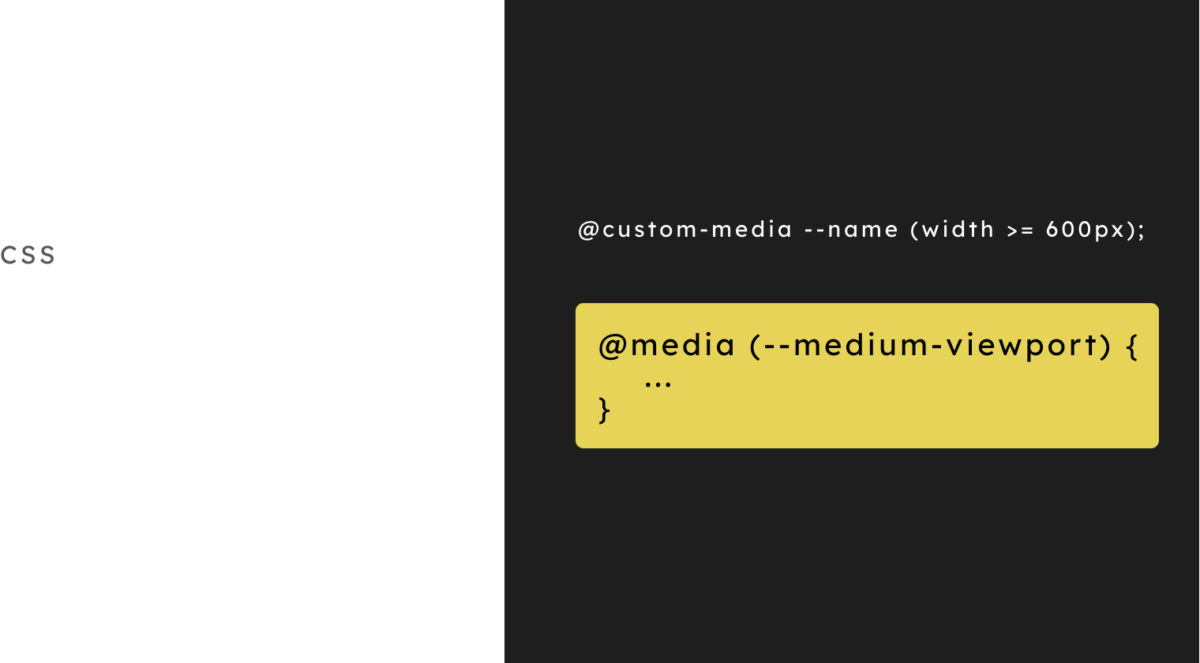
CSS Custom Media Queries
Learn CSS Custom Media Queries to efficiently manage and reuse media queries for responsive, modern web development
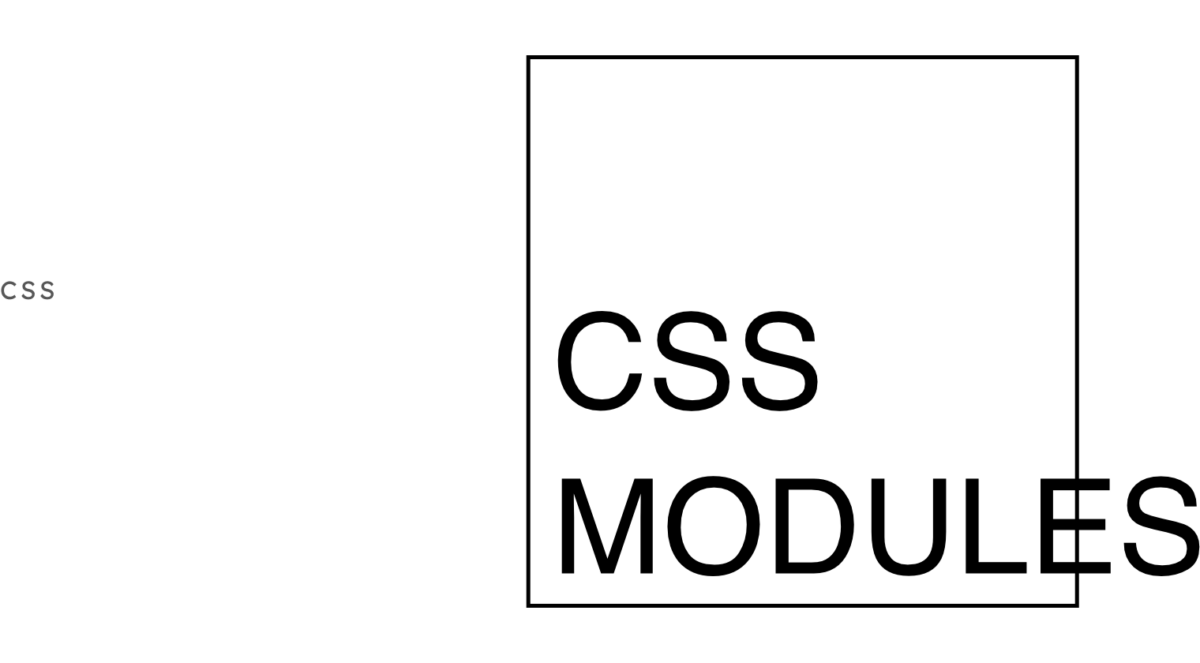
CSS Modules
Discover how CSS Modules combined with OOCSS/BEM enhance web development, offering a balanced approach to creating maintainable and streamlined UI.
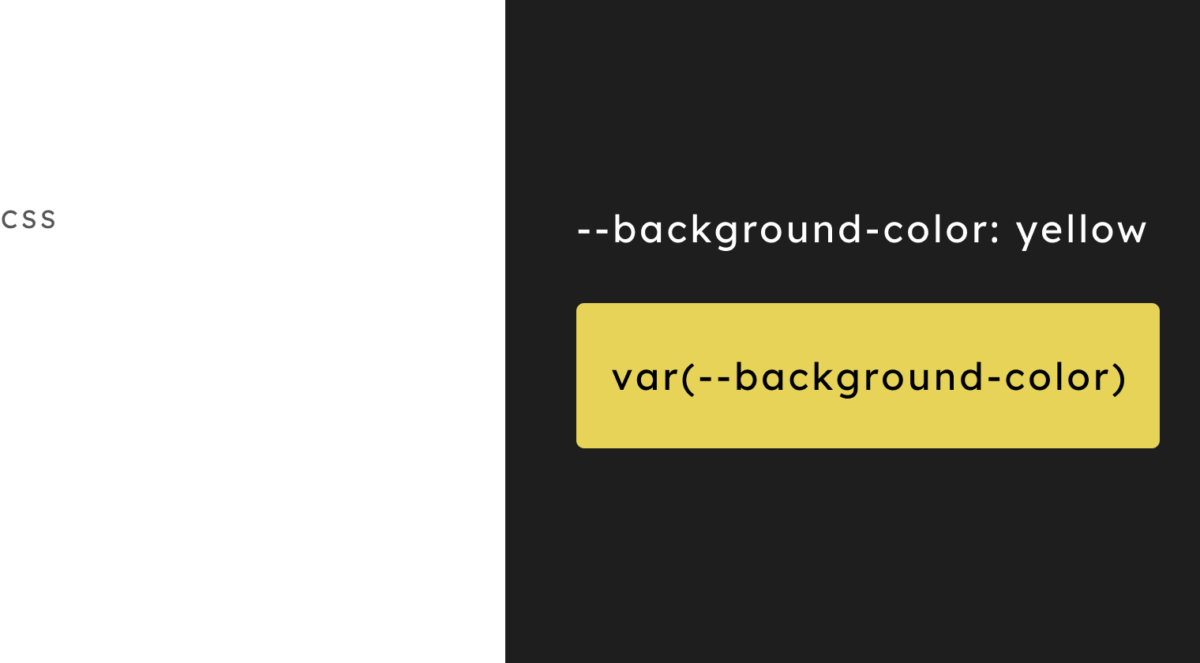
CSS Custom Properties & SASS variables
Unleash the power of CSS custom properties (aka. CSS variables) by comparing with SASS variables for enhanced styling flexibility and maintainability.
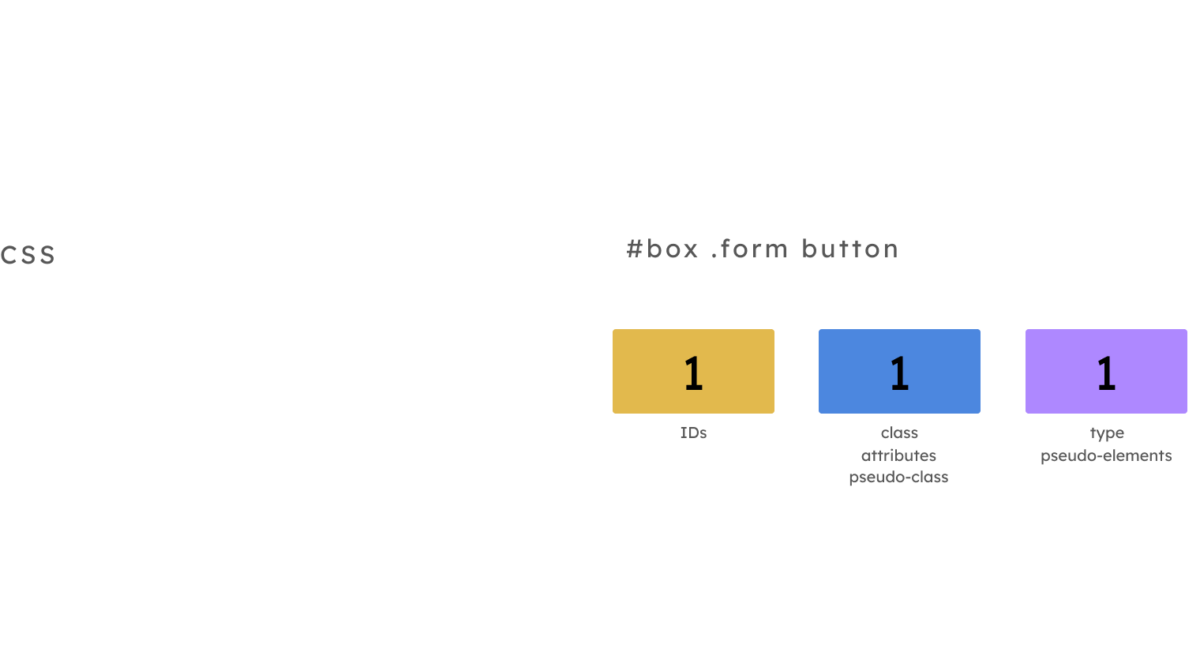
CSS Specificity in Depth
Learn how CSS specificity works and how to use it to write better CSS code and avoid CSS leak in this in-depth guide.
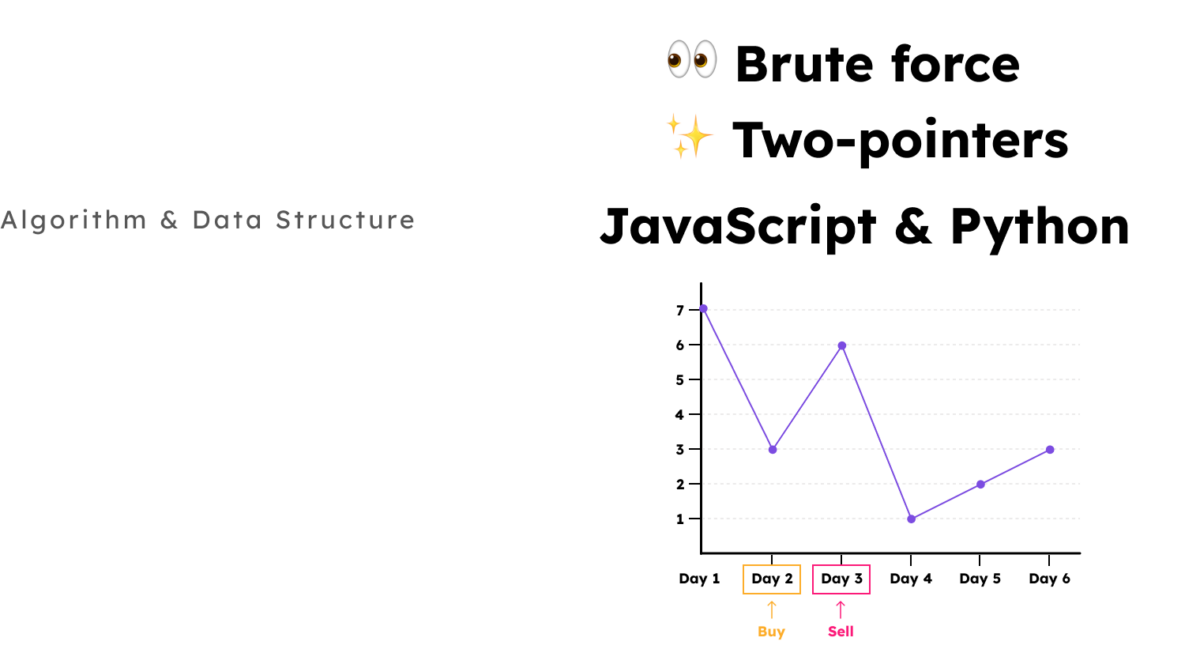
Best time to buy and sell stock (JavaScript and Python)
Discover how to determine the best buy and sell days to max profit from stock prices with solutions in both JS and Python.
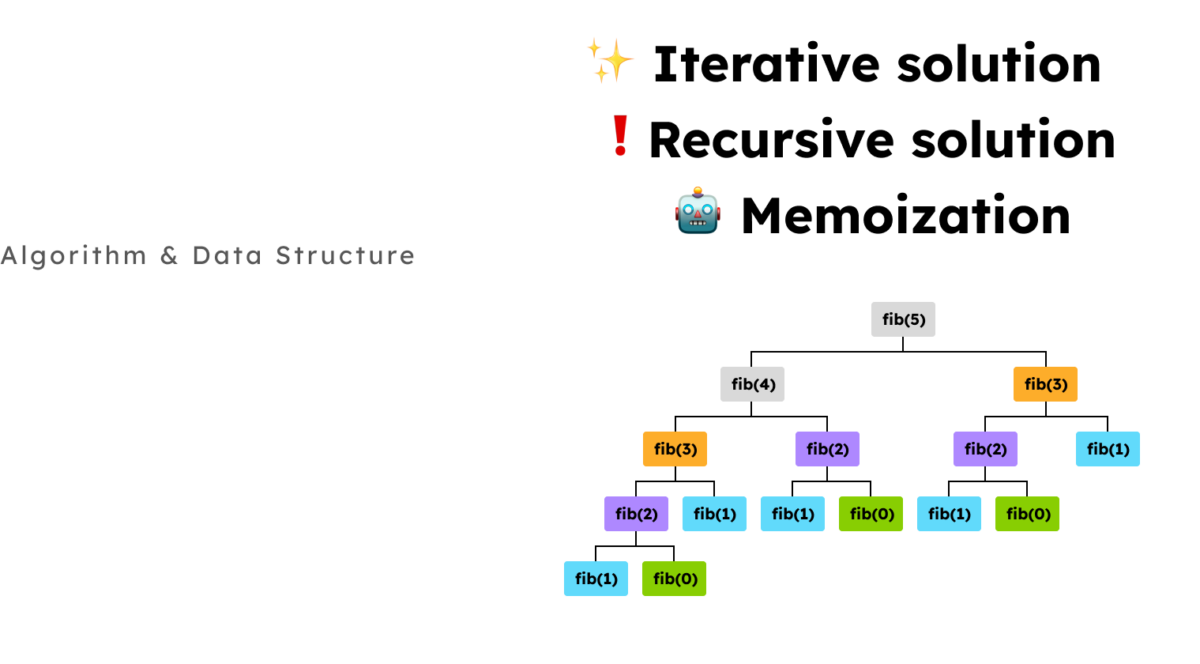
Nth Fibonacci Number
Discover how to calculate the Fibonacci sequence using both iterative and recursive approaches, and optimise recursion with memoization for improved efficiency.
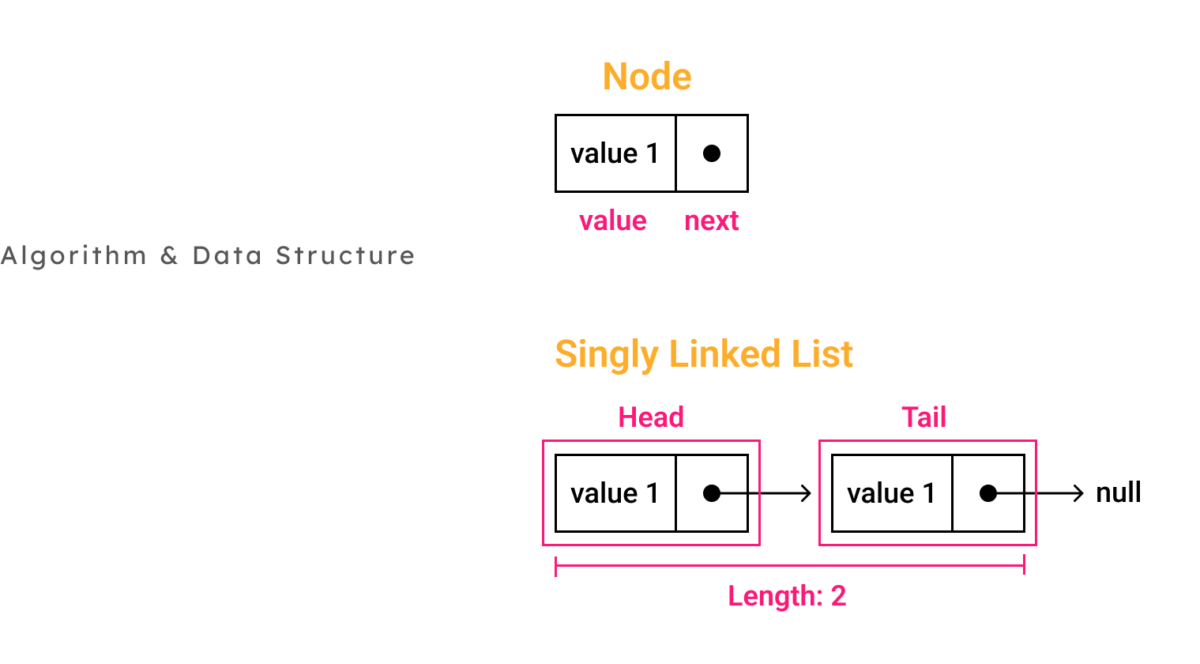
Singly Linked List & Array Methods
Dive into Singly Linked Lists: methods, complexities, and tech interview prep with this comprehensive guide.
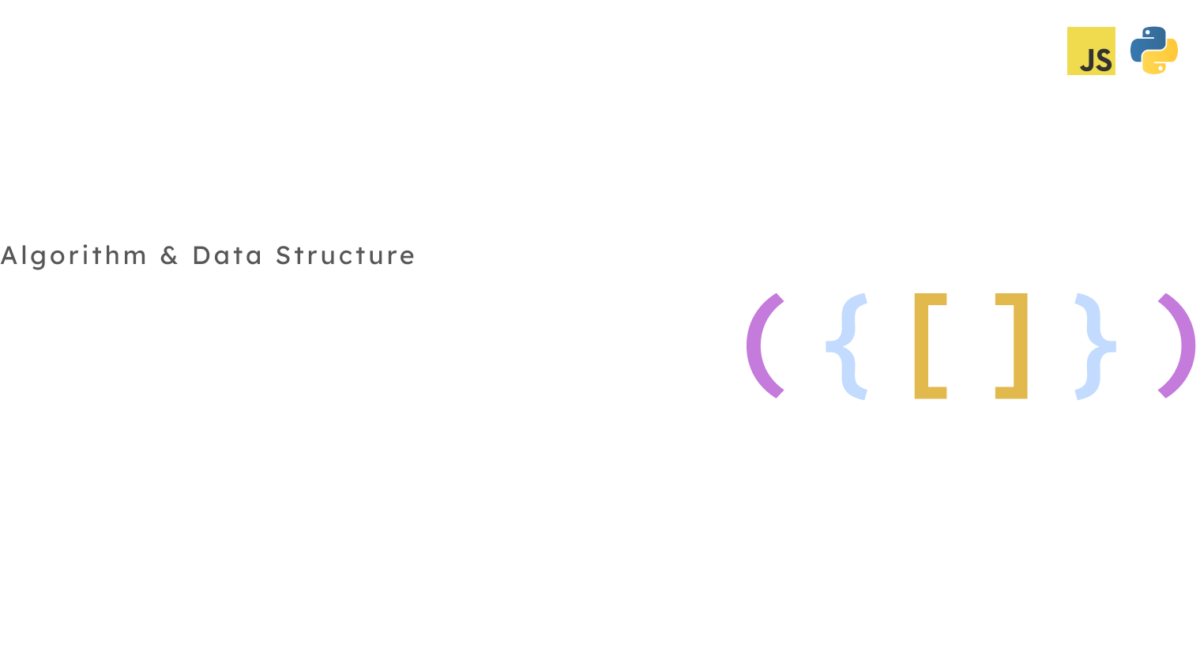
Valid Parentheses (JavaScript & Python)
Learn the ‘Valid Parentheses’ algorithm: Step-by-step guide in JavaScript (Python solution too) for your next interview
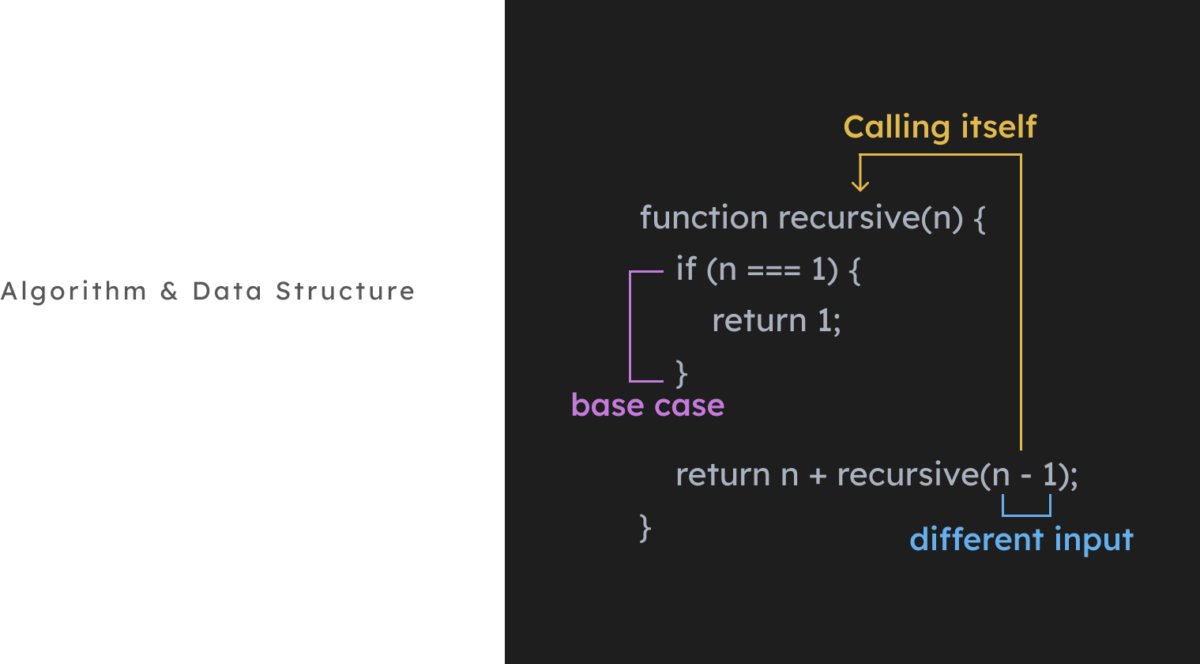
Recursion (with Common Interview Questions)
Learn recursion with clear examples with common interview questions and enhance your programming skills effectively.